C# mock interview practice on June 25, 2020
June 25, 202
Introduction
It took me less than 22 minute to finish code and also fixed the bug in my design as well. I like to write down my analysis and explain to myself how to solve the problem
Introduction
It took me less than 22 minute to finish code and also fixed the bug in my design as well. I like to write down my analysis and explain to myself how to solve the problem
Case study
Go over the example, how to find the target number, and which array should be dorminant one to get minimum rotations.
[3, 5, 1, 2, 3]
[3, 6, 3, 3, 4]
By my observation, I should find number 3, and also array B has 3 number 3.
I argue that the array should have a count of number from 1 to 6 at least half of (length + 1).
Go over the example, how to find the target number, and which array should be dorminant one to get minimum rotations.
[3, 5, 1, 2, 3]
[3, 6, 3, 3, 4]
By my observation, I should find number 3, and also array B has 3 number 3.
I argue that the array should have a count of number from 1 to 6 at least half of (length + 1).
But after the code submission, I failed one with more duplicate same number in another array. My argument is not correct. I have to consider all possibles and then get minimum one from all options.
Mock interview performance - beat 30%
I have to work on the first easy level algorithm, so my performance can be better to beat more people.
I have to work on the first easy level algorithm, so my performance can be better to beat more people.
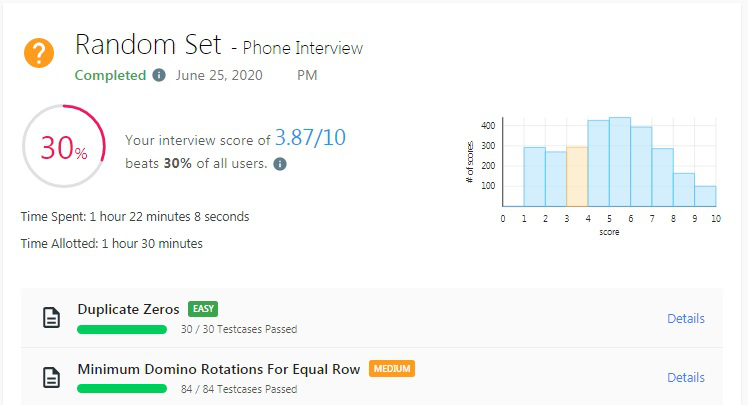
public class Solution {
public int MinDominoRotations(int[] A, int[] B) {
if(A == null || B == null || A.Length != B.Length || A.Length == 0)
return -1;
var length = A.Length;
var countA = new int[7];
var countB = new int[7];
for(int i = 0; i < length; i++)
{
countA[A[i]]++;
countB[B[i]]++;
}
var number = -1;
var foundA = false;
var foundB = false;
var candidates = new List<Tuple<char, int>>();
for(int i = 1; i < 7; i++)
{
if(countA[i] >= (length + 1)/ 2)
{
foundA = true;
number = i;
candidates.Add(new Tuple<char, int>('A', i));
break;
}
}
for(int i = 1; i < 7; i++)
{
if(countB[i] >= (length + 1)/ 2)
{
foundB = true;
number = i;
candidates.Add(new Tuple<char, int>('B', i));
break;
}
}
int min = length + 1;
foreach(var option in candidates)
{
var target = option.Item2;
var result = -1;
if(option.Item1 == 'A')
{
result = countMinimum(A, B, target);
}
else
{
result = countMinimum(B, A, target);
}
if(result != -1)
{
min = Math.Min(min, result);
}
}
return min == (length + 1)? -1 : min;
}
private int countMinimum(int[] A, int[] B, int number)
{
int minimumCount = 0;
for(int i = 0; i < A.Length; i++)
{
var current = A[i];
var isTarget = current == number;
if(!isTarget)
{
if(B[i] == number)
{
minimumCount++;
}
else
{
return -1;
}
}
}
return minimumCount;
}
}
No comments:
Post a Comment