C# mock interview 60 minutes practice on June 25 2020
June 25, 2020
Introduction
It is my first algorithm in mock interview on Leetcode.com. It took me almost 60 minutes to write code and made it work. I like to work on a case study, and then write down some notes for me to work on.
I have to prepare Facebook July 2020 phone screen. I like to work on 100 algorithms on Leetcode through Leetcode mock interview phone screen practice. So far, I only finished 6 algorithms.
Case study
Problem statement:
duplicate 0 in the array, and try to fit in the array in place from end of the array.
For example:
[1, 0] -> [1, 0]
[1, 0, 1] ->[1, 0, 0]
[1, 0, 0, 1] -> [1, 0, 0, 0]
Problem statement:
duplicate 0 in the array, and try to fit in the array in place from end of the array.
For example:
[1, 0] -> [1, 0]
[1, 0, 1] ->[1, 0, 0]
[1, 0, 0, 1] -> [1, 0, 0, 0]
The simple idea is to calculate how many numbers in the array can fit in the same array from left to right. Edge case is last number, it may be a zero, but only one position left for that zero.
I chose to take risk of index-out-of-range, I tried to cut number of variables to minimum, so the code can be clean and easy to read and test.
I added the comment to mark the bugs I made in my writing, and then I caught the issue through visual studio debugger. Every bug will take me a few minutes to fix, and I should think about better design in terms of definition of variables at the very beginning.
Mock interview performance - only beat 30%
I have to challenge myself to think about how to define variables more clearly defined.
I have to challenge myself to think about how to define variables more clearly defined.
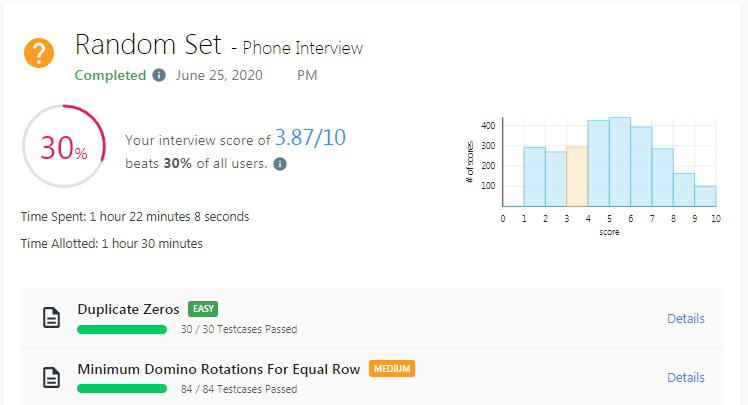
//[1,0]
public void DuplicateZeros(int[] numbers) {
if (numbers == null || numbers.Length == 0)
return;
var length = numbers.Length; //2 - bug 1
var next = 0;
var last = 0;
for (int i = 0; i < length && next < length; i++) // caught by debugger - length mixed with length + 1, what is good design? < or <=, think about more about common ways
{
var current = numbers[i];//0
// keep last i
last = i; // 0
if (current == 0)// true
{
next += 2; // 3
continue;
}
next += 1; // 1
}
int end = next - 1; // 3 should be next - 1, why the design needs -1 here? Not good design.
for (int i = last; i >= 0; i--) // last = 2
{
var current = numbers[i]; // 0
var isZero = current == 0;
if (!isZero)
{
numbers[end--] = numbers[i];
continue;
}
if (end > length - 1)
{
numbers[length - 1] = 0;
end -= 2; // caught by debugger - forget to take 2 off
}
else
{
numbers[end--] = 0;
numbers[end--] = 0;
}
}
}
No comments:
Post a Comment